In this post are some very simple codings that may be helpful in conjunction with some of the api codings later and some reviews of some important points that may be helpful in conjunction with some of the slightly more advanced api codings later
Simple 1 dimensional array to convenient Debug.Print
This very simply Function DBugPrntArr( ) makes a Debug.Print of the elements of a 1 dimensional array.
Here with a test example calling coding from here, https://eileenslounge.com/viewtopic....323516#p323516
https://www.excelfox.com/forum/showt...ll=1#post21746
Code:
Public Function DBugPrntArr(ByVal Arr As Variant) As Variant
'ReDim DBugPrntArr(LBound(Arr) To UBound(Arr))
Dim Var As Variant: ReDim Var(LBound(Arr) To UBound(Arr))
Dim Eye As Long, strOut As String
For Eye = LBound(Arr) To UBound(Arr)
Let Var(Eye) = Arr(Eye)
Let strOut = strOut & Arr(Eye) & ", "
Next Eye
Let strOut = "{" & Left(strOut, Len(strOut) - 2) & "}" ' Left(strOut, Len(strOut - 2)) is Take off last comma and space
Debug.Print strOut
'Stop ' Check watch window on var '
Let DBugPrntArr = Var
End Function
' Example to test
Sub arrChrs() ' https://eileenslounge.com/viewtopic.php?p=323516#p323516 https://www.excelfox.com/forum/showthread.php/2872-Appendix-Thread-App-Index-Rws()-Clms()-Majic-code-line-Codings-for-other-Threads-Tables-etc)-TEST-COPY?p=21746&viewfull=1#post21746
' 1b) test string example
Dim ZAC As String
Let ZAC = "ZAC" ' This is a demo example text string
Rem 2 String to array
Dim UniCrud As String: Let UniCrud = StrConv(ZAC, Conversion:=vbUnicode) ' "Z" & vbNullChar & "A" & vbNullChar & "C" & vbNullChar
Let UniCrud = Left(UniCrud, Len(UniCrud) - 1) ' "Z" & vbNullChar & "A" & vbNullChar & "C"
Dim Letas() As String: Let Letas() = Split(UniCrud, vbNullChar) ' { "Z" , "A" , "C" }
Call DBugPrntArr(Letas())
End Sub
The coding is principally just as a development aid to give a convenient visual output and one that can be copied easily , mostly for numbers, so characters, even if they are text, are not in the typically text required enclosed " " pair. In this example, for example, we get in the Immediate window
{Z, A, C}
Another useful similar function: ' This function assumes you have a 1 dimensional to fill from, and the array you fill to is a one dimensional array of the same first element indicie and that the array to fill is the same size or bigger
Code:
' This function assumes you have a 1 dimensional to fill from, and the array you fill to is a one dimensional array of the same first element indicie and that the array to fill is the same size or bigger
Public Function AddBytesToArray(ByVal arrTo As Variant, arrFrom As Variant) As Variant
Dim Cnt As Long
For Cnt = 0 To UBound(arrFrom)
Let arrTo(Cnt) = arrFrom(Cnt)
Next Cnt
Let AddBytesToArray = arrTo
End Function
Microsoft Unicode encoding UTF-16 LE
This is a quick review of how deep in memory windows typically holds text characters in a number code. The word code here meaning the sequence of 1 and 0 digits which represent any text character, (aka in the jargon, the encoding)
As example I choose a two character string.
The first character is capital A, which in almost all computer systems and conventions is assigned the capital number of 65
The second character is chosen as it has a to help show up the important characteristics of the UTF-16 LE Unicode encoding used by Microsoft.
Further more it happens to be a character that is both
_ assigned a decimal number (code point ) in Unicode (as almost all characters and everything in the world is, or will be eventually),
, but also
_ this character happens to be also assigned a decimal number (code point) in most of the windows code pages , ( which refer to characters code point in the range up to 255
This character I use as the second character example looks like 3 small dots, but is in fact, a single character which just pictorially looks like 3 small dots.
Just to demo that character, and how it looks compared to 3 normal dots , here are some different views of 5 characters comprising
[ 3 dots ( 3 standard dot characters ) ] [ a space ] [ the single character that looks like 3 small dots ]
... …
https://i.postimg.cc/MG5jBVKM/3-dots...n-in-Excel.jpg
https://i.postimg.cc/yNsZWvBC/3-dots...-VB-Editor.jpg
https://i.postimg.cc/vZWnQCJv/3-dots...ate-Window.jpg
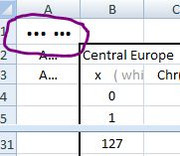
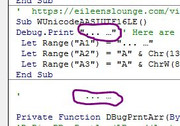
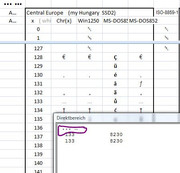
This simple coding gives us some outputs as discussed
Code:
' https://eileenslounge.com/viewtopic.php?p=297502#p297502 https://eileenslounge.com/viewtopic.php?p=297500#p297500 https://eileenslounge.com/viewtopic.php?p=323085#p323085 https://www.excelfox.com/forum/showthread.php/2824-Tests-Copying-Pasting-API-Cliipboard-issues-and-Rough-notes-on-Advanced-API-stuff?p=17883&viewfull=1#post17883
Sub WUnicodeAASIUTF16LE()
Debug.Print "... …" ' Here are 5 characters, 3 standard dots, a space and then the character which looks like 3 small dots
Let Range("A1") = "... …"
Let Range("A2") = "A" & Chr(133): Debug.Print Asc(Right(Range("A1").Value, 1)), AscW(Right(Range("A1").Value, 1))
Let Range("A3") = "A" & ChrW(8230): Debug.Print Asc(Right(Range("A2").Value, 1)), AscW(Right(Range("A2").Value, 1))
End Sub
An additional reason why I use this particular character, is that we avoid a typical awkward problem: We have the problem usually in the VB Editor / Immediate Window etc., when investigating Unicode characters, that the VB Editor / Immediate Window does not support most Unicode characters. This means we get an annoying ? or some incorrect character shown if we try to display it. However, if as in this character, … , the character does also appear in the code page, then usually the VB Editor / Immediate Window does show correctly the character, as seen in the last two screen shots
OK, so now we investigate how Microsoft holds those two characters: Follow careful these steps/ explanations:
_ The number representations are themselves wrapped inside
__ 4 bytes at the start holding the string length,
__ and two Bytes at the end both set at 0, ( which are together known as / representing the vbNullChar or or ChrW(0) , but this is not the number 0 which is almost always code point 48, Chr(48) , ChrW(48) )
We are less concerned here with those start and end Bytes, - rather we are interested principally here on the Bytes representing the characters, or rather how we represent the numbers, (decimal code points ) assigned to the characters
_ We need the decimal code points (decimal numbers) :
__ For the character capital A it is 65 ;
__For demonstration purposes I will choose the Unicode number for … which is 8230
_ Each of the two Bytes is 8 bits, and each bit can be 0 or 1 – so in other words, 8 bit Binary or B bit base 2, or 8 digit binary or 8 digit base 2
The maximum number possible for each Byte will therefore be the binary
11111111
which is
2^7 + 2^6 + 2^5 + 2^4 + 2^3 + 2^2 + 2^1 + 2^0
= 128 + 64 + 32 + 16 + 8 + 4 + 2 + 1
= 255
What we do is use the two Byte pieces, like a 2 digit base 256 number system that is "the wrong way around", or the other way around to what we may be more familiar with the base 2 (binary ) number system, whose first two bits or pieces are
2^1 2^0
0-1 0-1
, so we are looking at a system using two pieces (Bytes) like this
256^0 256^1
0-255 0-255
In this system, a number up to 255 is easy to see the representation, The total value is
low-end + 256 x high-end .
So for our A for example we have
65 0
For our 8230 it needs a bit more maths: The total value is again low-end + 256 x high-end.
8230 can be written as 256 * 32 + 38
The high-end bye is 8230 \ 256 = 32
The low-end byte is 8230 Mod 256 = 38
So Windows writes it as
38 32
Here a short coding for those two characters and another , e , whose decimal code point is mist usually 101. The coding uses the function discussed at the start of this post and the main Calling coding uses the Byte type (array) ideas of the previous post
Code:
Sub ByteArray()
Dim Harry() As Byte '
Let Harry() = "A" & ChrW(8230) & "e" ' https://eileenslounge.com/viewtopic.php?p=297329#p297329
Call DBugPrntArr(Harry()) ' {65, 0, 38, 32, 101, 0}
End Sub
Bookmarks