Make UserForm(s) object of the type / class ufResults
Very important to remember at this point is that we have no "thing" or object, just some notes / sketches of how we would like an object to be. We need to talk about making an object from those notes / sketches, what we refer to as something like Instantiating, instanciating, Initialising, making an object fro a class.
We won’t go here into the exact details of all the different ways to do this, but they are similar to the ideas of referring to some external libraries, as discussed here for example https://www.excelfox.com/forum/showt...ll=1#post10568
https://www.excelfox.com/forum/showt...ll=1#post10567
Strictly speaking an external Library is a package that can include amongst other things Classes to provide functionality of some sort of another. But in end effect we want the same thing: An object variable which can then be used in such a form
___= ThatLibraryObject.StufffromItIWant
Our class module so far is nothing more than a blank pop up ( a UserForm is basically a pop up , sometimes called a Form )
There is not a lot we can do with an object made from it, and an object made form it similarly can’t do much.
One thing we can do is get it to pop up. Another thing we can do is programmatically change the caption on a made object.
So this next simple coding :
_ makes an object from the class, ufResults, and the variable objufResults will be pointing to it
_ Shows that User form
_ Changes the caption to New Caption
As this is "real" coding, it can go in any existing object code module, or a normal code module. https://i.postimg.cc/nrHyDfsT/Real-c...mal-module.jpg
(It shouldn’t go in anything to do with the class module ufResults , or any other class module, as you can not run coding from a bit of paper….. )
Code:
Option Explicit
Sub ufTest() ' https://www.excelfox.com/forum/showthread.php/2965-Class-related-Stuff-Userforms?p=24186&viewfull=1#post24186
Rem 1 making an instance of ufResults
Dim objufResults As ufResults
Set objufResults = New ufResults
Rem 2 The object exist, and could be considerd perhaps to have a similar status to a worksheet, although we do not have a corresponding code module for it, but we can imagine one looking like the ufResults class module
objufResults.Show vbModeless ' vbModeless means I means I can do anything outside the UserForm whilst its open, and also that the code moves on. (If I used vbModeless, then I would effectively "hang" at this code line until I closed the UserForm )
Application.Wait Time:=Now + TimeValue("00:00:03")
Rem 3 I am changing the caption on the current
Let objufResults.Caption = "New Caption" ' This changes the name in the current objufResults
Application.Wait Time:=Now + TimeValue("00:00:03")
Rem 4 Close the userform instance, (checking the number of loaded userforms before and after)
Debug.Print UserForms.Count ' 1
Unload Object:=objufResults ' close the user form instance objufResults
Debug.Print UserForms.Count ' 0
End Sub
Important to note is that after running the coding, the caption seen in the class module, ufResults is as initially. We should expect this: That caption name, UserForm1 , is what is given every time we make an instance of ufResults.
https://i.postimg.cc/gJPFGZmC/User-F...ass-module.jpg
UserForm1 remains the caption name in the Form diagram in the class module.jpg
_._________________________
Here is the quirk ###
This is almost the same coding as the last, coding, and on running it, exactly the same thing happens
Code:
Sub ufTest2()
Rem 1 making an instance of ufResults
'Dim ufResults As ufResults
' Set ufResults = New ufResults
Rem 2 The object does not exist ?????
ufResults.Show vbModeless
Application.Wait Time:=Now + TimeValue("00:00:03")
Rem 3 I am changing the caption on the current
Let ufResults.Caption = "New Caption"
Application.Wait Time:=Now + TimeValue("00:00:03")
Rem 4 Close the userform instance, (checking the number of loaded userforms before and after)
Debug.Print UserForms.Count
Unload Object:=ufResults
Debug.Print UserForms.Count
End Sub
I have made just two main coding changes.
_ I have changed the name of the variable which was pointing to the object ,in all the corresponding places in the coding, from objufResults to ufResults, (which remains our class name as well)
, and
_ I have removed the coding that instantiates an object from the class, ( I have ' commented out Rem 1)
By the way if I put that (Rem 1 coding back in, then once again on running it, exactly the same thing happens . This latter statement is perhaps understandable, (although it is generally regarded to be unwise to use the same name for different things and can cause unexpected problems )
What is less understandable is how the coding should work fine when I appear to not instantiate the class, that is to say make an instance of the class. In most coding of this nature we would receive an error saying something like the object variable, (in this second coding ufResults, does not exist when we first try to use it , ( in this coding at code line ufResults.Show vbModeless )
So what is going on???
As Rory said…. Unlike normal classes, UserForms are auto-instantiating, so you can simply call them by (class) name and a new instance of the class is created. ….. as you use ufResults for the first time, a new instance of the form is created.
It's confusing because you effectively get a variable (pointing to the object instantiated from the class) of the same name as the class. ###
Whilst this may be considered convenient, it could easily be one reason why many people do not understand the subtle point about class, and may miss altogether that they are dealing with a class for the case of a UserForm, in particular as many people are only interested in a single instance of any particular UserForm type, and on top of this, they may not be interested particularly in changing the class name, as we did.
So they insert a UserForm module, for example a single first one. It gets the default name UserForm1. Then they go off happily in coding towards the start of the coding doing things like, pseudo coding,
UserForm1.DoSomething
They may well believe they are dealing with an object variable, UserForm1, from the start, and indeed think they have been exclusively dealing with an object, or object related things, fir example whilst working with the UserForm module and it’s various tools . But that is not the case. They where dealing with class things, and then the first time they used the word UserForm1 they effectively had this coding going on
Dim UserForm1 As UserForm1
Set UserForm1 = New UserForm1
UserForm1.DoSomething
, where UserForm1 is the class, and UserForm1 is the object
Code:
Option Explicit
Sub ufTest3() ' https://www.excelfox.com/forum/showthread.php/2965-Class-related-Stuff-Userforms?p=24186&viewfull=1#post24186
Rem 1 making an instance of ufResults - we can do this, or it will be done automatically by first attempt to use UserForm1 where an object is expected
'Dim UserForm1 As UserForm1
' Set UserForm1 = New UserForm1
Rem 2 The object does not exist , but will when the code line is done
UserForm1.Show vbModeless
Application.Wait Time:=Now + TimeValue("00:00:03")
Rem 3 I am changing the caption on the current
Let UserForm1.Caption = "New Caption"
Application.Wait Time:=Now + TimeValue("00:00:03")
Rem 4 Close the userform instance, (checking the number of loaded userforms before and after)
Debug.Print UserForms.Count ' 1
Unload Object:=UserForm1
Debug.Print UserForms.Count ' 0
End Sub
https://i.postimg.cc/wjNGmyb0/class-...User-Form1.jpg
class UserForm1 and object variable UserForm1.jpg 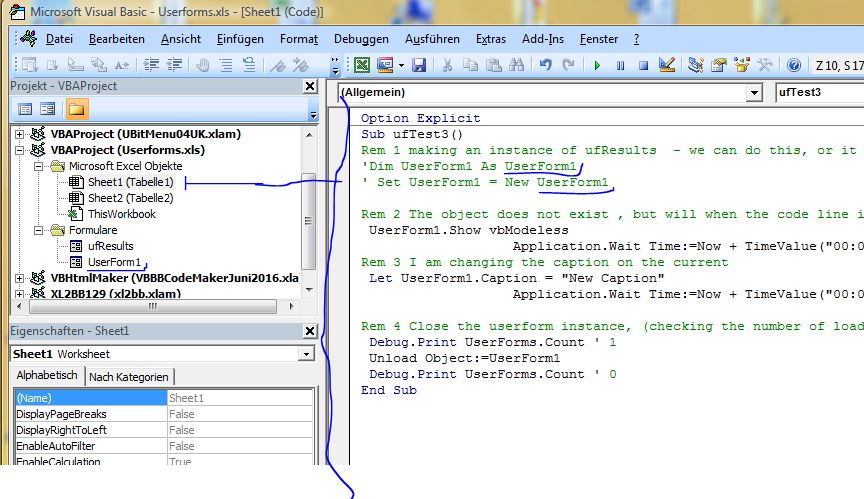
_._______
In the next post we will do just a very simple example of a userform with some coding "in it"
Bookmarks